# Let's dive into advanced Ruby on Rails testing with MiniTest and RSpec!
Rails makes it easy to write robust tests. Whether you prefer MiniTest or RSpec, you've got great options for unit, integration, and system tests.
I remember when I first started with Rails, testing felt overwhelming. But once I got the hang of it, I couldn't imagine developing without a solid test suite. It gives you so much confidence in your code.
Let's start with MiniTest since it comes built-in with Rails. Here's a basic unit test:
class UserTest < ActiveSupport::TestCase
test "should not save user without email" do
user = User.new
assert_not user.save, "Saved the user without an email"
end
end
Pretty straightforward, right? We're just checking that a user can't be saved without an email. But we can get fancier:
class UserTest < ActiveSupport::TestCase
test "should generate api key on create" do
user = User.create(email: "[email protected]", password: "password")
assert_not_nil user.api_key
assert_equal 32, user.api_key.length
end
end
This test ensures our User model is generating an API key when created. We're not just checking for presence, but also the expected length.
Now let's look at an integration test:
class UserFlowsTest < ActionDispatch::IntegrationTest
test "can create an account and login" do
# Create account
post "/users", params: { user: { email: "[email protected]", password: "password" } }
assert_response :redirect
follow_redirect!
assert_select "div.alert", "Account created successfully!"
# Login
post "/login", params: { email: "[email protected]", password: "password" }
assert_response :redirect
follow_redirect!
assert_select "h1", "Welcome, [email protected]!"
end
end
This test simulates a user creating an account and then logging in. It's checking both the server responses and the rendered HTML.
System tests take this even further, allowing you to interact with your app like a real user:
class SignupTest < ApplicationSystemTestCase
test "creating a new account" do
visit root_path
click_on "Sign Up"
fill_in "Email", with: "[email protected]"
fill_in "Password", with: "password"
fill_in "Password confirmation", with: "password"
click_on "Create Account"
assert_text "Welcome, [email protected]!"
end
end
This test actually opens a browser (usually headless) and interacts with your app. It's as close as you can get to manual testing, but automated!
Now, let's switch gears to RSpec. While not built-in, many Rails devs prefer its more expressive syntax. Here's a unit test:
RSpec.describe User, type: :model do
it "is not valid without an email" do
user = User.new(password: "password")
expect(user).to_not be_valid
end
it "generates an api key on create" do
user = User.create(email: "[email protected]", password: "password")
expect(user.api_key).to be_present
expect(user.api_key.length).to eq(32)
end
end
Notice how it reads almost like English? That's one of the things I love about RSpec.
For integration tests, RSpec uses request specs:
RSpec.describe "User flows", type: :request do
it "allows creating an account and logging in" do
# Create account
post "/users", params: { user: { email: "[email protected]", password: "password" } }
expect(response).to redirect_to(root_path)
follow_redirect!
expect(response.body).to include("Account created successfully!")
# Login
post "/login", params: { email: "[email protected]", password: "password" }
expect(response).to redirect_to(dashboard_path)
follow_redirect!
expect(response.body).to include("Welcome, [email protected]!")
end
end
And for system tests:
RSpec.describe "Signup process", type: :system do
it "allows a new user to sign up" do
visit root_path
click_on "Sign Up"
fill_in "Email", with: "[email protected]"
fill_in "Password", with: "password"
fill_in "Password confirmation", with: "password"
click_on "Create Account"
expect(page).to have_content "Welcome, [email protected]!"
end
end
Both MiniTest and RSpec offer powerful features for more advanced testing scenarios. Let's explore some:
Mocking and stubbing are crucial for isolating the code you're testing. Here's how you might stub an API call in MiniTest:
def test_fetch_user_data
stub_request(:get, "https://api.example.com/users/1")
.to_return(status: 200, body: { name: "John Doe" }.to_json)
user_data = UserService.fetch_user_data(1)
assert_equal "John Doe", user_data[:name]
end
And in RSpec:
it "fetches user data" do
allow(HTTParty).to receive(:get)
.with("https://api.example.com/users/1")
.and_return({ "name" => "John Doe" })
user_data = UserService.fetch_user_data(1)
expect(user_data[:name]).to eq("John Doe")
end
Both frameworks also support data factories for generating test data. With FactoryBot, which works with both MiniTest and RSpec:
FactoryBot.define do
factory :user do
email { Faker::Internet.email }
password { "password" }
trait :admin do
admin { true }
end
end
end
Now you can easily create test users:
user = FactoryBot.create(:user)
admin = FactoryBot.create(:user, :admin)
This is super helpful for setting up complex test scenarios without cluttering your test files.
Another advanced technique is using shared examples or contexts. In RSpec:
RSpec.shared_examples "requires authentication" do
it "redirects to login page if user is not authenticated" do
get described_path
expect(response).to redirect_to(login_path)
end
end
RSpec.describe "Protected routes" do
describe "GET /dashboard" do
let(:described_path) { dashboard_path }
it_behaves_like "requires authentication"
end
describe "GET /settings" do
let(:described_path) { settings_path }
it_behaves_like "requires authentication"
end
end
This allows you to reuse common test scenarios across multiple contexts.
As your test suite grows, you'll want to keep an eye on performance. Both MiniTest and RSpec support parallel testing, which can significantly speed up your test runs:
# In config/environments/test.rb
config.active_job.queue_adapter = :test
config.active_job.queue_adapter.perform_enqueued_jobs = true
# In your test file
class ParallelTest < ActiveSupport::TestCase
parallelize(workers: :number_of_processors)
end
For RSpec, you can use the parallel_tests gem.
Remember, tests are more than just a safety net - they're documentation for your code. Well-written tests can serve as a guide for other developers (or future you) to understand how your code is supposed to work.
I hope this deep dive into Rails testing has been helpful! Whether you choose MiniTest or RSpec, the important thing is to write tests consistently. Start small, focus on the critical paths in your application, and gradually build up your test suite. Before you know it, you'll have a robust set of tests that give you the confidence to refactor and add new features without fear of breaking things.
Happy testing!
ruby
Mastering Rails Testing: From Basics to Advanced Techniques with MiniTest and RSpec
Rails testing with MiniTest and RSpec offers robust options for unit, integration, and system tests. Both frameworks support mocking, stubbing, data factories, and parallel testing, enhancing code confidence and serving as documentation.
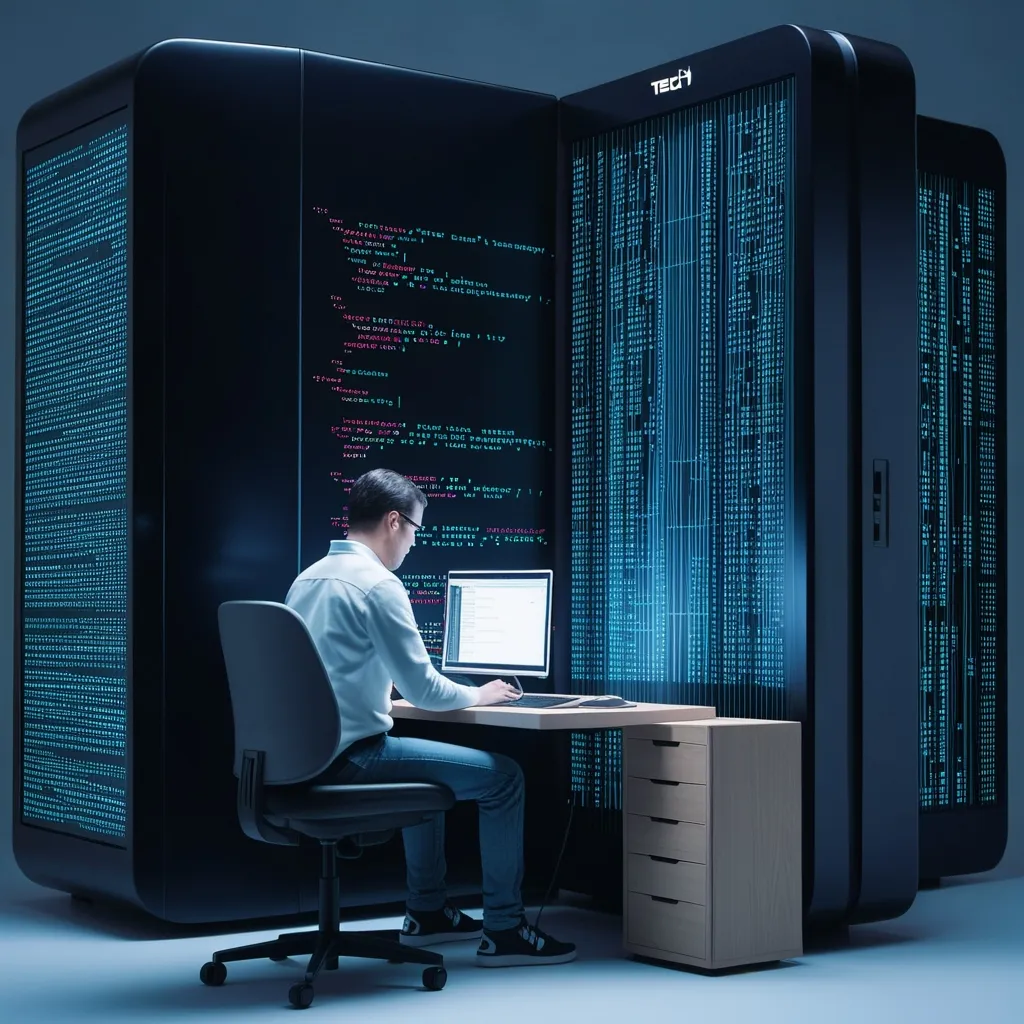